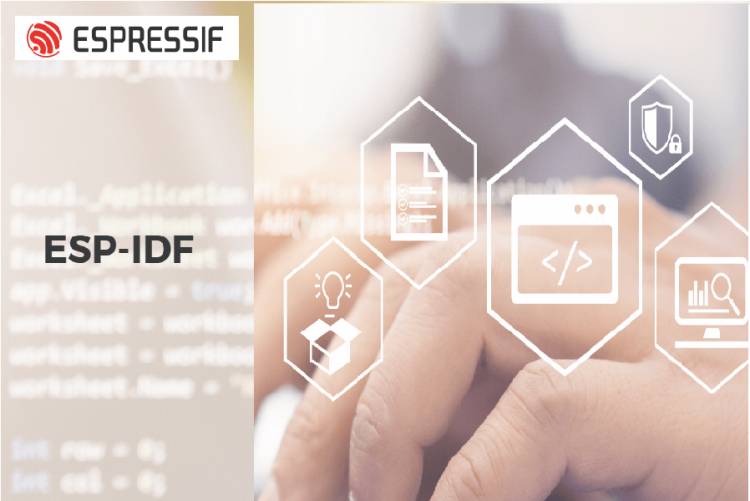
What is ESP-IDF?
ESP-IDF (Espressif IoT Development Framework)
ESP-IDF (Espressif IoT Development Framework) is an IoT (Internet of Things) software development framework developed by Espressif Systems. ESP-IDF is designed specifically for Espressif's WiFi and Bluetooth-enabled microcontrollers such as the ESP32 and ESP32-S2. This framework includes tools, libraries, and documentation used to develop applications on these microcontrollers.
ESP-IDF is designed to make best use of the features of microcontrollers equipped with features such as low power consumption, high performance, WiFi and Bluetooth connections. It is written in C to provide developers with low-level hardware control and is customizable and extensible.
ESP-IDF comes with a large collection of APIs, sample projects, debugging tools and documentation. It also includes a set of tools used to compile, install and debug users' projects. ESP-IDF is very popular as a framework often used in the development of ESP32-based projects.
ESP-IDF is Espressif's official IoT Development Framework for the ESP32, ESP32-S, ESP32-C and ESP32-H series SoCs. It provides a self-contained SDK for any general application development on these platforms using programming languages such as C and C++.
Below is an example C code:
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "freertos/task.h"
#include "esp_wifi.h"
#include "esp_log.h"
//Set the necessary information to connect to the WiFi network here
#define WIFI_SSID "your_wifi_ssid"
#define WIFI_PASSWORD "your_wifi_password"
static const char *TAG = "wifi_example";
void wifi_init_sta() {
// WiFi connection configuration
wifi_init_config_t cfg = WIFI_INIT_CONFIG_DEFAULT();
esp_wifi_init(&cfg);
wifi_config_t wifi_config = {
.sta = {
.ssid = WIFI_SSID,
.password = WIFI_PASSWORD,
},
};
esp_wifi_set_config(WIFI_IF_STA, &wifi_config);
esp_wifi_start();
}
void app_main() {
//start WiFi connection
wifi_init_sta();
while (1) {
vTaskDelay(1000 / portTICK_RATE_MS);
ESP_LOGI(TAG, "Hello, ESP32-C3 is connected to WiFi!");
}
}
ESP-IDF does not have an official integrated development environment (IDE), but is often used on top of Visual Studio Code (VSCode), powered by Espressif. Therefore, when working with ESP-IDF you may often need to use a text editor followed by Visual Studio Code or another IDE.
To use Visual Studio Code with ESP-IDF, you can follow these steps:
1. Download and Install Visual Studio Code: Download Visual Studio Code from the official website of Visual Studio Code and install it on your computer.
2. Add ESP-IDF Plugin to Visual Studio Code: You may need to add the "PlatformIO" plugin, which is a plug-in for ESP-IDF, to Visual Studio Code. Open Visual Studio Code, go to the "Extensions" section on the left side, then you can add it by searching for the "PlatformIO IDE" extension and installing it.
3. Download and Install ESP-IDF: Download ESP-IDF from the Espressif IDF GitHub repository and install it on your computer by following the instructions. This usually includes Python and other dependencies.
4. Create Project and Open with Visual Studio Code: Create a project to work with ESP-IDF. Next, open Visual Studio Code and open your project with this IDE. This process provides Visual Studio Code's capabilities to edit and compile your project with ESP-IDF integration.
ESP-IDF has components and APIs that can also be used with high-level languages such as Python and JavaScript. In particular, the "idf.py" command line tool, a Python-based tool, can be used to manage and compile ESP-IDF projects.
In general, most uses of ESP-IDF are based on the C language, but integration with high-level languages is also possible in certain cases. Using the C language is widely preferred, especially due to the requirements of microcontroller programming and its advantages in low-level hardware control.
More detailed information:
https://www.espressif.com/en/products/sdks/esp-idf