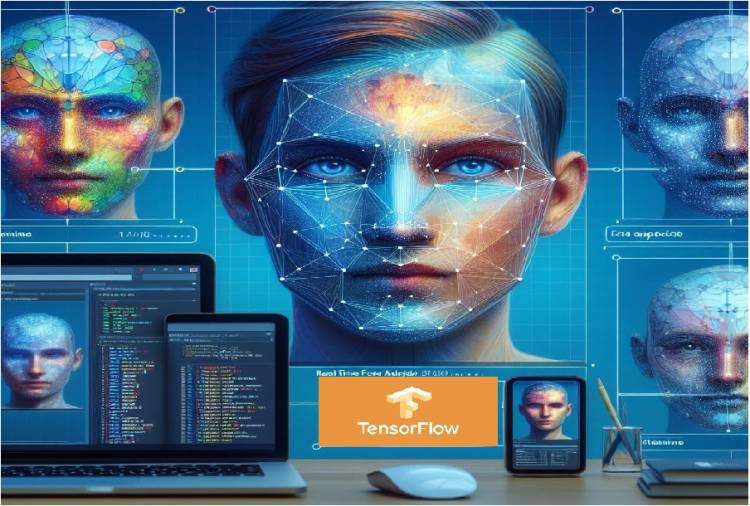
Real-Time Face Analysis with TensorFlow.js: Structure, Usage and Application Areas
TensorFlow.js is a JavaScript library used to build and run machine learning and deep learning models in web browsers and the Node.js environment. This article will discuss the structure of TensorFlow.js, how and why it is used, and specifically a code example in a real-time facial analysis application.
What is TensorFlow.js?
TensorFlow.js is the JavaScript version of Google's open source machine learning library TensorFlow. It can be used both in the browser and in the Node.js environment. TensorFlow.js makes it easy to build learning models based on user interactions in web-based applications and in the browser.
How and Why to Use?
TensorFlow.js provides a set of tools for building, training, and deploying machine learning and deep learning models. JavaScript provides users with a powerful toolset for running and training complex models in browser-based applications. This allows users to create training sets from their browser and quickly apply models across a variety of application domains.
Real-time Face Analysis with TensorFlow.js: The example that will be discussed in this article targets real-time face analysis using TensorFlow.js and the FaceMesh model.
Below is a code example:
// Loading the necessary libraries
<script src="https://cdn.jsdelivr.net/npm/@tensorflow/tfjs"></script>
<script src="https://cdn.jsdelivr.net/npm/@tensorflow-models/facemesh"></script>
// Camera settings and model loading
async function setupCamera() {
const video = document.getElementById('inputVideo');
const stream = await navigator.mediaDevices.getUserMedia({ 'video': true });
video.srcObject = stream;
return new Promise((resolve) => {
video.onloadedmetadata = () => {
resolve(video);
};
});
}
// Loading the FaceMesh model
async function loadFacemesh() {
return await facemesh.load();
}
// Face detection and updating results
async function detectFace(video, facemeshModel) {
const predictions = await facemeshModel.estimateFaces(video);
updateResults(predictions);
requestAnimationFrame(() => detectFace(video, facemeshModel));
}
//Updating the results
function updateResults(predictions) {
const faceResultsElement = document.getElementById('faceResults');
if (predictions.length > 0) {
faceResultsElement.innerHTML = `<p>Face detected!</p>`;
} else {
faceResultsElement.innerHTML = `<p>No face detected.</p>`;
}
}
// Starting the application
async function run() {
const video = await setupCamera();
const facemeshModel = await loadFacemesh();
detectFace(video, facemeshModel);
}
run();
This code allows the user camera access and performs real-time facial analysis using TensorFlow.js and the FaceMesh model.
Application Areas: TensorFlow.js' implementation of real-time facial analysis can be used in a number of application areas:
Face detection and tracking in video conferencing applications.
Facial recognition and access control in security applications.
User interaction and expression analysis in games.
Trying products on online shopping platforms in virtual trial rooms.
TensorFlow.js provides JavaScript developers with a powerful toolset to build machine learning applications. The real-time facial analysis example demonstrates the application versatility and ease of use of TensorFlow.js, which gives JavaScript developers a significant advantage for creating interactive and user-oriented applications.
You can visit the Site for Sample Application: ai.milivolt.news
Related article: https://milivolt.news/post/integration-of-programming-languages-with-tensorflow