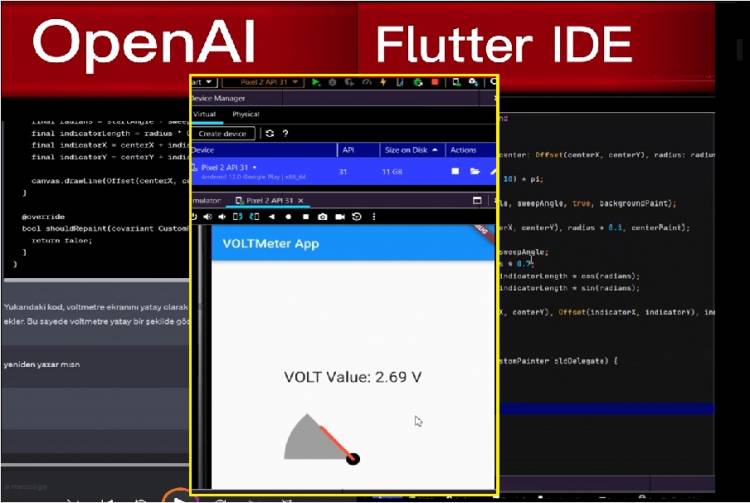
Developing Mobile Applications with Artificial Intelligence – Voltmeter Interface Application
In this article, we will talk about an application we made with OpenAI.
We wanted to make an application with Flutter-Dart. We got help from OpenAI while coding.
This application is a graphical interface application. We thought that a microcontroller system was set up for volt measurement. We wanted to show how the digital data coming to the mobile application via Bluetooth or Wifi module is visualized.
We have previously made bluetooth and wifi applications on our site and youtube channel. Among our Bluetooth applications, we have shown how to receive data from an MCU system with a mobile application.
Link: Measuring DC Volts with Android Phone - Voltmeter
In our current application, numerical values (volt values) are generated randomly. Every 2 seconds, these numerical data are reflected on the graphic screen.
Flutter-Dart codes are below. These codes are easily understandable when examined block by block.
import 'dart:async';
import 'dart:math';
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text('Horizontal Voltmeter Application'),
),
body: Center(
child: VoltmeterApp(),
),
),
);
}
}
class VoltmeterApp extends StatefulWidget {
@override
_VoltmeterAppState createState() => _VoltmeterAppState();
}
class _VoltmeterAppState extends State<VoltmeterApp> {
double _voltageValue = 0.0;
Timer? _timer;
@override
void initState() {
super.initState();
_startTimer();
}
@override
void dispose() {
_timer?.cancel();
super.dispose();
}
void _startTimer() {
// Starts a timer that produces random voltage values at certain intervals.
_timer = Timer.periodic(Duration(seconds: 2), (timer) {
final randomValue = Random().nextDouble() * 10; // random voltage value between 0 and 10
setState(() {
_voltageValue = randomValue;
});
});
}
@override
Widget build(BuildContext context) {
//Formats the voltage value as a two-digit fraction.
final formattedVoltage = _voltageValue.toStringAsFixed(2);
return Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text(
'Voltage Value: $formattedVoltage V',
style: TextStyle(fontSize: 24),
),
SizedBox(height: 20),
Voltmeter(_voltageValue),
],
);
}
}
class Voltmeter extends StatelessWidget {
final double voltage;
Voltmeter(this.voltage);
@override
Widget build(BuildContext context) {
return Center(
child: SizedBox(
width: 200,
height: 100, // Decreases the height for horizontal display.
child: Row(
children: [
Transform.rotate(
angle: pi, // Flips the graph horizontally.
child: CustomPaint(
painter: VoltmeterPainter(voltage),
),
),
],
),
),
);
}
}
class VoltmeterPainter extends CustomPainter {
final double voltage;
VoltmeterPainter(this.voltage);
@override
void paint(Canvas canvas, Size size) {
final centerX = size.width / 2;
final centerY = size.height / 2;
final radius = min(centerX, centerY);
final backgroundPaint = Paint()
..color = Colors.grey
..style = PaintingStyle.fill;
final centerPaint = Paint()
..color = Colors.black
..style = PaintingStyle.fill;
final indicatorPaint = Paint()
..color = Colors.red
..style = PaintingStyle.fill
..strokeCap = StrokeCap.round
..strokeWidth = 10.0;
final rect = Rect.fromCircle(center: Offset(centerX, centerY), radius: radius);
final startAngle = 0; // Sets the starting angle to 0.
final sweepAngle = (voltage / 10) * pi;
canvas.drawArc(rect, startAngle, sweepAngle, true, backgroundPaint);
canvas.drawCircle(Offset(centerX, centerY), radius * 0.1, centerPaint);
final radians = startAngle + sweepAngle;
final indicatorLength = radius * 0.7;
final indicatorX = centerX + indicatorLength * cos(radians);
final indicatorY = centerY + indicatorLength * sin(radians);
canvas.drawLine(Offset(centerX, centerY), Offset(indicatorX, indicatorY), indicatorPaint);
}
@override
bool shouldRepaint(covariant CustomPainter oldDelegate) {
return false;
}
}